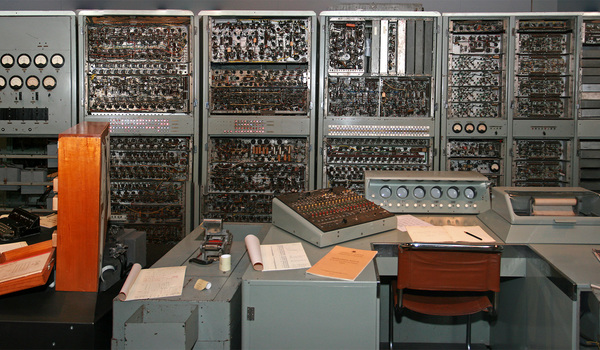
Specific Things to RememberApril 18, 2014
This will be a list (that I will hopefully update frequently) of specific issues I've run into/things I want to remember so I don't have to go digging around for how to solve them again in the future.
- Don't use Heroku if you have any special binary dependencies beyond ImageMagick. Definitely don't try to use their Vulcan build server (which is now deprecated), it gave wildly inconsistent compiling results. Use a VPS like Digital Ocean.
- To compile ffmpeg follow these instructions. Also just remember that sometimes you have to recompile the ffmpeg dependencies (last time I had to recompile H.264) to get it to build successfully. God that whole process was such a headache.
- In Rails 4 the images in the Vendor folder are no longer included automatically in the asset pipeline in production. This means the you have to add this line to config/environments/production.rb:
config.assets.precompile += %w(*.png *.jpg *.jpeg *.gif)
If you have the choice put them in /app or /public. Remember this for Spree where the CSS files have to be in /vendor but any images you add do not. Then make sure to follow the advice below on referencing images.
- If you reference images that are in the Asset Pipeline in a CSS file you have to reference them with erb. Add a .erb extension to the end of your stylesheet and then use asset_path to make sure it's pointing to the correct image name after assets are compiled:
background: url(<%= asset_path 'rails.png' %>");
- If you create aliases in .bashrc on a linux server, you not only need to restart the server, but also the shell. Otherwise you can use:
. ~/.bashrc
to reload the .bashrc file in your current shell. - To access regex captures you can use square bracket lookup notation. [0] returns the entire regex as a string, [1] returns first capture, [2] the second capture, etc:
"foo bar".match(/(foo)\s(bar)/)[1]
=> "foo" - When using Nokogiri to parse through an HTML/XML document, if you need to locate an element based on some unusual attribute other than class/id like 'title', you can do this using the format 'element[attribute="value"]'. So in a scraper using Mechanize and Nokogiri you might type:
page.parser.at_css('span[title="description"]')
and it would locate the first <span> element with a title attribute that has the value "description" - I know the NOT operator is a very simple concept, but I always seem to forget about it when I need a conditional that checks if a string does not include some text, and often I just do this using the non-match regex oprator (!~). While this works great I think the following is cleaner:
!'Foo Bar'.include?('Baz')
Very obvious to most people I know, but I just wanted to note it down as a reference. - Learn the various rails conventions. Model names are singular:
rails g model User
class User < ActiveRecord::Base
end
Controller names are plural:rails g controller Users
class UsersController < ApplicationController
end
The rails resource generator is a great way to quickly generate the needed model, controller, routes, and migration necessary to create a new Rails resourcerails g resource Person name:string
Migrations should be written as:rails g migration AddPartNumberToProducts
- Some postgres notes. psql. "\list" or "\l" lists all databases, "\connect DB_NAME" or "\c" connects to a specific database, "\dt" lists all tables in current db, and "ALTER TABLE mytable ADD COLUMN mycolumn character varying" creates a new text column in the specified table. "d+ table_name" can be used to list all columns in a given table.
- When working with multiple heroku apps attached to one local repo, connect to existing repos be creating a git remote:
git remote add heroku_production git@heroku.com:project-production.git
git remote add heroku_test git@heroku.com:project-test.git
Then if you need to execute heroku commands, just use the --app option to specify which app you are trying to interact with:heroku pgbackups:capture --app project-production
One instance that took me a minute to realize was when using curl to download the latest postgres db backup, you need to include the --app option inside of the backticks:curl -o staging.dump `heroku pgbackups:url --app project-test`
To apply a dump:pg_restore -O -d database_name file.dump
- In the rails console, to output data in YAML format just type 'y ' before the code. Also typing an underscore '_' references the previously returned line.
- When there's an issue with javascript check the browser console (Firefox gave more insightful feedback). Since all of the javascript files are compiled into one, if there's an error in the code of any file it can break all of the javascript functionality on the page.
- When setting up Paypal Express Checkout there are two types of API credentials, Signature Credentials and Certificate Credentials. Only one can be activated in a PayPal account at a time (Spree uses Signature).
- When using render and defining an object, the object will be assigned to a variable with the same name as the partial so:
render partial: 'posts/post', object: @user_post
will assign the @user_post object to the variable 'post'. A more verbose alternative is specifying local variables with:render partial: 'posts/post', :locals => {:user_posting => @user_post}
which allows you to define things more explicitly and include more than one variable. - To allow a rails model (ex. Brand) to update an associated model (ex. Product) Brand must include:
accepts_nested_attributes_for :product
- To browse heroku file system use:
heroku run bash
- To use view helper methods inside of a controller use:
view_context.method
- redis-cli notes:
redis-cli monitor - A debugging command that streams back every command processed by the Redis server.
info keyspace - lists keyspaces, a concept somewhat similar to databases.
keys * - lists all keys in current keyspace
to access keys you must know the type of the value which you can get with:
type key
you can then use the correct get method depending on the type:
for "string": get key
for "hash": hgetall key
for "list": lrange key 0 10 - the last two ints represent the index range (exclusive)
for "set": smembers key
for "zset": zrange key 0 10 - the last two ints represent the index range (inclusive) - UFW
ufw show added
will show current rules prior to enabling - To identify a process opening too many files use:
lsof -n | sed -E 's/^[^ ]+[ ]+([^ ]+).*$/\1/' | uniq -c | sort | tail
which lists the PID on the right and the number of files it has open on the left. Then you can useps -p 1234 -o comm=
replacing 1234 with your PID to get info about the program that's causing the issue - To do a partial search just with Postgres use LIKE in a where query:
Country.where('name LIKE :search', search: '%United%')
- To search by filename:
find /path/to/folder -name '*query*'